[spring] 테스트 코드 By starseat 2023-01-31 15:32:36 java/spring Post Tags # 테스트 코드 - 단위테스트(Unit Test) : TDD의 첫 번째 단계인 **기능 단위의 테스트 코드 작성** - TDD(Test Driven Development) - 테스트 주도 개발. - 테스트 코드를 먼저 작성 - 참고: [TDD 실천법과 도구" 책 PDF](https://repo.yona.io/doortts/blog/issue/1) - 레드 그린 사이클 - Red: 항생 실패하는 테스트 코드 먼저 작성 - Green: 테스트가 통과하는 프로덕션 코드 작성 - Refactor: 테스트가 통과하면 프로덕션 코드를 리팩토링 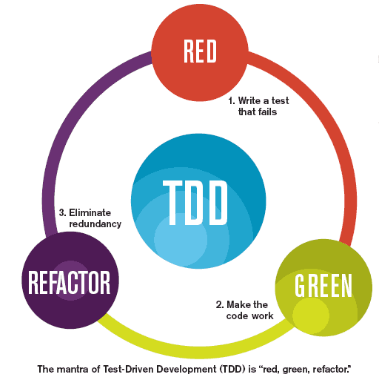 ## 단위 테스트 코드 작성 이점 - 개발 단계 초기에 문제를 발견할 수 있음 - 개발자가 나중에 코드를 리팩토링하거나 라이브러리 업그레이드 등에서 기존 기능이 올바르게 작동하는지 확인 가능 (예: 회귀 테스트) - 기능에 대한 불확실성 감소 - 시스템에 대한 실제 문서 제공 - 즉, 단위 테스트 자체가 문서로 사용할 수 있음 (feat. [RestDoc](https://www.google.com/search?q=spring+boot+rest+docs&rlz=1C1YTUH_koKR1027KR1027&sxsrf=AJOqlzU0FpvqaLC8v2GW5yocQ1O8hPmqXQ%3A1675146522911&ei=GrXYY-CqN6il1e8PlumZuAY&oq=spring+boot+rest+doc&gs_lcp=Cgxnd3Mtd2l6LXNlcnAQARgAMgUIABCABDIFCAAQgAQyBQgAEIAEMgUIABCABDIFCAAQgAQyCAgAEIAEEMsBMgQIABAeMgQIABAeMgQIABAeMgQIABAeOgQIIxAnOgcIABCABBAKOgQIABADOgsIABCABBCxAxCDAToRCC4QgAQQsQMQgwEQxwEQ0QM6DQguELEDEMcBENEDEEM6DgguEIAEELEDEMcBENEDOgQIABBDSgQIQRgASgQIRhgAUABYsxhg0ChoAHAAeACAAYYBiAH5EJIBBDIuMTiYAQCgAQHAAQE&sclient=gws-wiz-serp)) - 기존 기능이 잘 작동되는 것을 보장 ## 테스트 프레임워크 - xUnit 이 있음. - 개발환경(x)에 따라 Unit 테스트를 도와주는 도구 - 대표적인 xUnit 프레임워크 - **JUnit - java** - DBUnit - DB - CppUnit - C++ - NUnit - .net # 테스트 코드 샘플 - 테스트 코드 예제는 [[spring] 테스트 코드 - 예제 코드](https://starseat.net/blog/view/135) 에 작성하고 있음. ## Controller ```java import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest; import org.springframework.boot.context.junit4.SpringRunner; import org.springframework.boot.test.web.servlet.MockMvc; import org.springframework.boot.test.web.servlet.ResultActions; import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status; @RunWith(SpringRunner.class) // 1 @WebMvcTest(controllers = HomeController.class) // 2 public class HomeControllerTest { @Autowired // 3 private MockMvc mvc; // 4 @Test public void hellTest() throws Exception { String hello = "hello"; mvc.perform(get("/hello")) // 5 .andExpect(status().isOk()) // 6 .andExpect(content().string(hello)); // 7 } } ``` 1.`@RunWith(SpringRunner.class)` - 테스트 진행 시 JUnit에 내장된 실행자 외에 다른 실행자 실행. - 스프링 부트 테스트와 JUnit 사이의 연결자 역할 2.`@WebMvcTest` - Web(Spring MVC) 용 - 선언할 경우 @Controller, @ControllerAdvice 등 사용 가능 - 단 @Service, @Component, @Repository 등은 사용할 수 없음 - 이 테스트 코드는 컨트롤러만 사용하므로 선언 3.`@Autowired` - 스프링이 관리하는 빈(Bean) 주입 받음 4.`private MockMvc mvc;` - 웹 API 테스트 용 - 스프링 MVC 테스트의 시작 점 - HTTP 의 GET, POST 등에 대한 API 테스트 가능 5.`mvc.perform(get("/hello"))` - MockMvc 를 통해 "/hello" 주소로 HTTP GET 요청 6.`.andExpect(status().isOk())` - mvc.perform 의 결과 검증 - HTTP Header 의 Status 검증 (200, 404, 500 등의 상태 검증) 7.`.andExpect(content().string(hello))` - mvc.perform 의 결과 검증 - 응답 본문 내용 검증 # 참조 - [스프링 부트와 AWS로 혼자 구현하는 웹 서비스](https://jojoldu.tistory.com/463) Previous Post [spring] build.gradle 기초 정리 Next Post [spring] 자주 사용하는 어노테이션 (작성 중)